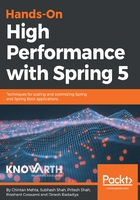
Caching
In order to improve the performance of an application, caching heavy operations is inevitable. Spring 3.1 added a great abstraction layer called caching that helped to abandon all custom-implemented aspects, decorators, and code injected into the business logic related to caching.
Spring applies caching to the methods of Spring beans using AOP concepts; we learned about it in the AOP concepts section of this chapter. Spring creates proxies of the Spring beans where the methods are annotated to be cached.
In order to leverage the benefits of Spring's caching abstraction layer, just annotate heavy methods with @Cacheable. Also, we need to notify our application that methods are cached by annotating our configuration class with @EnableCaching. The following is the example of caching a method:
@Cacheable("accounts")
public Account findAccountById(int accountId){
The @Cacheable annotation has the following attributes:
- value: Name of cache
- key: Caching key for each cached item
- condition: Defines whether to apply caching or not based on the evaluation of the Spring Expression Language (SpEL) expression
- unless: This is another condition written in SpEL, and if it is true, it prevents the return value from being cached
The following are additional annotations provided by Spring related to caching:
- @CachePut: It will let the method execute and update the cache
- @CacheEvict: It will remove stale data from the cache
- @Caching: It allows you to group multiple annotations @Cacheable, @CachePut, and @CacheEvict on the same method
- @CacheConfig: It will allow us to annotate our entire class instead of repeating on each method
We can use @Cacheable on methods that are retrieving data and use @CachePut on a method that performs insertion to update the cache. The code sample is as follows:
@Cacheable("accounts" key="#accountId")
public Account findAccountById(int accountId){
@CachePut("accounts" key="#account.accountId")
public Account createAccount(Account account){
Annotating methods to cache the data would not store the data; for that, we need to implement or provide CacheManager. Spring, by default, provides some cache managers in the org.springframework.cache package and one of them is SimpleCacheManager. The CacheManager code sample is as shown:
@Bean
public CacheManager cacheManager() {
CacheManager cacheManager = new SimpleCacheManager();
cacheManager.setCaches(Arrays.asList(new
ConcurrentMapCache("accounts"));
return cacheManager;
}
Spring also provides support to integrate the following third-party cache managers:
- EhCache
- Guava
- Caffeine
- Redis
- Hazelcast
- Your custom cache