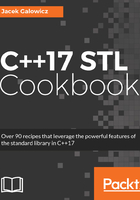
上QQ阅读APP看书,第一时间看更新
How to do it...
In this section, we will use the two different ways to access an std::vector, and then see how we can utilize them to write safer programs without decreasing readability.
- Let's include all the needed header files, and fill an example vector with 1000 times the value 123, so we have something we can access:
#include <iostream>
#include <vector>
using namespace std;
int main()
{
const size_t container_size {1000};
vector<int> v (container_size, 123);
- Now, we access the vector out of bounds using the [] operator:
cout << "Out of range element value: "
<< v[container_size + 10] << '\n';
- Next, we access it out of bounds using the at function:
cout << "Out of range element value: "
<< v.at(container_size + 10) << '\n';
}
- Let's run the program and see what happens. The error message is GCC specific. Other compilers would emit different but similar error messages. The first read succeeds in a strange way. It doesn't lead the program to crash, but it's a completely different value than 123. We can't see the output line of the other access because it purposefully crashed the whole program. If that out of bounds access was an accident, we would catch it much earlier!
Out of range element value: -726629391
terminate called after throwing an instance of 'std::out_of_range'
what(): array::at: __n (which is 1010) >= _Nm (which is 1000)
Aborted (core dumped)