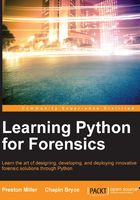
Libraries
Libraries, or modules, expedite the development process, making it easier to focus on the intended purpose of our script rather than developing everything from scratch. External libraries can save large amounts of developing time, and, if we're being honest, they are often more accurate and efficient than any code we can cobble together. There are two types of library: standard and third-party libraries. Standard libraries are distributed with every installation of Python and carry commonly used code supported by the software foundation. Third-party libraries introduce new code and add or improve functionality to the standard Python installation.
Installing third-party libraries
We know that we do not need to install standard modules because they come with Python, but what about third-party modules? The Python Package Index is a great place to start looking for third-party libraries at https://pypi.python.org/pypi. This service allows tools such as pip
to install packages automatically. If an Internet connection is not available or the package is not found on PyPi, a setup.py
file can usually be used to install the module manually. The examples of using pip
and setup.py
are shown later. Tools such as pip
are very convenient as they handle the installation of dependencies. Check whether items are already installed and suggest upgrades if an older version is installed. An Internet connection is required to check for online resources, such as dependencies and newer versions of a module. However, pip
can also be used to install code on an offline machine.
These commands are run in the terminal or command prompt, not the Python interpreter. Please note that in the example mentioned later, full paths may be necessary if your Python executable is not included in the current environment's PATH
variable. Pip must be run from an elevated console, either using sudo
or an elevated Windows command prompt. Full documentation for pip
can be found at http://pip.pypa.io/en/stable/reference/pip/:
$ pip install flexx Downloading/unpacking flexx Downloading flexx-0.1.tar.gz (109kB): 109kB downloaded Running setup.py (path:/private/tmp/pip_build_root/flexx/setup.py) egg_info for package flexx Installing collected packages: flexx Running setup.py install for flexx Successfully installed flexx Cleaning up... $ git clone https://github.com/williballenthin/python-registry.git $ cd python-registry $ python setup.py install running install ...[Installation Log]... Installed /Library/Python/2.7/site-packages/enum34-1.0.4-py2.7.egg Finished processing dependencies for python-registry==1.1.0
Libraries in this book
In this book, we use many third-party libraries that can be installed with pip or the setup.py
method. However, not all third-party modules can be installed so easily and sometimes require searching the Internet. As you may have noted in the previous code block, some third-party modules, such as the python-registry module, are hosted on a source code management system such as GitHub. GitHub and other SCM services allow us to access publicly available code and view changes made to it over time. Alternatively, code can sometimes be found on a blog or a self-hosted website. In this book, we will provide instructions on how to install any third-party modules we use.
Python packages
A Python package is a directory containing Python modules and the __init__.py
file. When we import a package, the __init__.py
code is executed. This file contains imports and code required to run other modules in the package. These packages can be nested, and they exist within subdirectories. For example, the __init__.py
file can contain import statements that bring in each Python file in the directory and all of the available classes or functions when the folder is imported. The following is an example directory structure and the __init__.py
file that shows how the two interact when imported. The last line in the following code block imports all specified items in the subDirectory
's __init__.py
file.
The hypothetical folder structure is as follows:
| -- packageName/ | -- __init__.py | -- script1.py | -- script2.py | -- subDirectory/ | -- __init__.py | -- script3.py | -- script4.py
The top-level __init__.py
file contents are as follows:
from script1 import * from script2 import FunctionName from subDirectory import *
The code mentioned later executes the __init__
script mentioned previously, and it will import all functions from script1.py
, only FunctionName
from script2.py
, and any additional specifications from subDirectory/__init__.py
:
import packageName