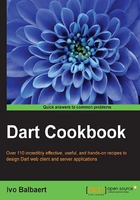
Parsing command-line arguments
A server app that runs a batch job often takes parameter values from the command line. How can we get these values in our program?
How to do it...
The obvious way to parse command-line arguments is as follows (see command_line_arguments.dart
):
void main(List<String> args) { print("script arguments:"); for(String arg in args) print(arg); }
Now, the command dart command_line_arguments.dart param1 param2 param3
gives you the following output:
script arguments:
param1
param2
param3
However, you can also test this from within Dart Editor, open the menu Run, and select Manage Launches (Ctrl + Shift + M). Fill in the parameters in the Script arguments window:

Script arguments
What if your parameters are in the key:value
form, as shown in the following code?
par1:value1 par2:value2 par3:value3
In this case, use the following code snippet:
for(String arg in args) { List<String> par = arg.split(':'); var key = par[0]; var value = par[1]; print('Key is: $key - Value is: $value'); }
The previous code snippet gives you the following output:
Key is: par1 - Value is: value1
Key is: par2 - Value is: value2
Key is: par3 - Value is: value3
The split
method returned List<String>
with a key and value for each parameter. A more sophisticated way to parse the parameters can be done as follows:
final parser = new ArgParser(); argResults = parser.parse(args); List<String> pars = argResults.rest; print(pars); // [par1:value1, par2:value2, par3:value3]
Again, use split
to get the keys and values.
How it works...
The main()
function can take an optional argument List<String> args
to get parameters from the command line. It only takes a split of the parameter strings to get the keys and values.
The second option uses the args
package from the pub repository, authored by the Dart team. Include args:any
in the dependencies section of the pubspec.yaml
file. Then, you can use the package by including import 'package:args/args.dart';
at the top of the script. The args
package can be applied both in client and server apps. It can be used more specifically for the parsing of GNU and POSIX style options and is documented at https://api.dartlang.org/apidocs/channels/stable/dartdoc-viewer/args/args.
See also
- Refer to the Searching in files recipe in Chapter 6, Working with Files and Streams, for an example of how to use the args package with a flag