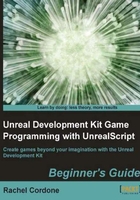
Time for action – Creating config variables
- To let the game know that our class needs to save config variables, first we need to let it know which file to use.
class AwesomeActor extends Actor placeable config(Game);
This tells the game that our class' config variables will be defined in the Game ini files as opposed to Engine or Input and so on.
- Now, let's make a config variable.
var config int MyConfigInt;
Config vars can have parentheses to let level designers change them, but they can NOT be put in the default properties block. Doing so will give a compiler error. Instead, we define their default properties in the INI file we specified. Since we used
Game
, we would put the default inDefaultGame.ini
. Let's open that up now. - In
DefaultGame.ini
we can see a bunch of different sections, starting with a line surrounded by brackets. The inside of these brackets specifies the package and class that the section is defining defaults for, like this:[Package.Class]
- In our case our package name is
AwesomeGame
, and the class we need to define isAwesomeActor
. At the end ofDefaultGame.ini
, make a new section surrounded by brackets.[AwesomeGame.AwesomeActor]
- Right after that we can define any default values we need.
MyConfigInt=3
Once we're done, our section should look like this:
[AwesomeGame.AwesomeActor] MyConfigInt=3
- Let's see if it works! In
AwesomeActor.uc
, changePostBeginPlay
to logMyConfigInt
.var config int MyConfigInt; function PostBeginPlay() { 'log("MyConfigInt:" @ MyConfigInt); }
- Compile and run, then check the log file.
[0008.66] ScriptLog: MyConfigInt: 3
Have a go hero – Editable configurable variable?
Knowing what you know about different ways to define default values for variables, what do you think would take precedence: The config file or a value set by the level editor? Try adding a variable that's both configurable and editable and logging the result.
What just happened?
If we look in UDKGame.ini
, we can see that the variable has shown up there as well. Remember that the UDK.ini
files are built from the Default.ini
files, and instead of changing the Default.ini
files, the player and the game work with the UDK.ini
ones. That way the game always has a fail safe with the Default.ini
files. If the player or a setting menu in the game changed MyConfigInt
to 5
for example, then the player changed their mind and used a settings menu to reset everything to the default value, we would be able to do that by using the Default.ini
value for that variable.
Now that we've learned about the different types of variables and ways to set their values, let's take a look at some common operators we can use on our variables.